MATLAB
MATLAB (matrix laboratory) is a multi-paradigm numerical computing environment and fourth-generation programming language. Developed by MathWorks, MATLAB allows matrix manipulations, plotting of functions and data, implementation of algorithms, creation of user interfaces, and interfacing with programs written in other languages, including C, C++, Java, and Fortran.
Versions
We currently offer the following MATLAB versions:
math/MATLAB/2017a
math/MATLAB/2018b
math/MATLAB/2021b
You can query the versions yourself with module av matlab
.
Usage
Load the most recent version available with:
module load math/MATLAB
You can also load a particular version with module load math/MATLAB/<version>
, e.g. module load math/MATLAB/2017a
See the vendor documentation: https://www.mathworks.com/help/matlab/
MATLAB Version 2021b
If the Help Improve MATALAB feature is enabled, MATLAB returns an error on exit
if part of the MATLAB preferences system was not initialized before exit.
E.g.:
>> exit(0)
Error using exit
Unrecognized function or variable 'Settings'.
To work around this issue, you can ensure that the settings are initialized properly by calling:
settings
in your MATLAB code. Alternatively, you can uncheck the Improve MATLAB by sending user experience information to MathWorks
option under Preferences -> MATLAB -> General.
Toolboxes
The currently available toolboxes are
Toolbox | Version |
---|---|
Curve Fitting Toolbox | 3.5.8 |
Econometrics Toolbox | 5.1 |
Fuzzy Logic Toolbox | 2.4 |
Image Acquisition Toolbox | 5.5 |
Image Processing Toolbox | 10.3 |
MATLAB Compiler | 7.0 |
MATLAB Compiler SDK | 6.6 |
Mapping Toolbox | 4.7 |
Optimization Toolbox | 8.2 |
Parallel Computing Toolbox | 6.13 |
Partial Differential Equation Toolbox | 3.1 |
Signal Processing Toolbox | 8.1 |
Statistics and Machine Learning Toolbox | 11.4 |
Symbolic Math Toolbox | 8.2 |
Wavelet Toolbox | 5.1 |
MATLAB Java Crash Dump Files
If you want to prevent MATLAB from spamming your home directory with the java.log.<number>
files, you can set the following environment variable:
export MATLAB_LOG_DIR=~/Path/To/Your/Directory
You can also define this environment variable in your .bashrc
file.
Using Matlab
Using Matlab is possible several ways, which we would like to briefly introduce here.
Examples
were mostly taken from the vendor documentation
Matlab Startup Options
Starting MATLAB from a Linux system prompt using matlab
gives you several start-up options. We only present an overview of the most commonly used options. A complete list can be found in the MATLAB documentation.
Option | Result |
---|---|
-nojvm | Start MATLAB without the JVM software. Features that require Java are not supported. |
-nodisplay | Start the JVM software without starting MATLAB desktop. Does not display X commands. Overrides the DISPLAY environment variable. |
-nosplash | Do not display the splash screen during startup. |
-singleCompThread | Limit MATLAB to a single computational thread. For numerical computations only. (Default) |
-multiCompThread | Do not limit MATLAB to a single computational thread. |
-sd folder | Set the MATLAB folder, specified as string, e.g. -sd "~/matlab" sets the folder to matlab. |
-r statement | Execute the statement. Use this option for interactive workflows. Do not use this option with the -batch option. |
-batch statement | Execute MATLAB script, statement, or function non-interactively. Important features are: -nodesktop , -nosplash , logs to stdout & stderr. |
-batch *statement*
is to be preferred to -r *statement*
.Interactive Job Submission
Request resources according to your needs, e.g. with:
srun -n<ntasks> -p<partition> -A<account> -t<time> --mem<amount> --pty --preserve-env $SHELL
Load the desired Matlab module:
module load math/MATLAB
Now you can run Matlab. Add -nojvm
flag to start Matlab without the Java virtual machine, -nosplash
prevents Matlab from displaying the Matlab logo.
matlab -nodisplay -nosplash
Check the version info and the available toolboxes for that version:
>> ver
gives:
-------------------------------------------------------------------------------------------
MATLAB Version: 9.5.0.944444 (R2018b)
...
Operating System: Linux 3.10.0-957.5.1.el7.x86_64 #1 SMP Fri Feb 1 14:54:57 UTC 2019 x86_64
Java Version: Java is not enabled
-------------------------------------------------------------------------------------------
MATLAB Version 9.5 (R2018b)
Curve Fitting Toolbox Version 3.5.8 (R2018b)
Econometrics Toolbox Version 5.1 (R2018b)
...
The use of the Matlab-Compiler is to be preferred!
Only a limited number of licences are available on the cluster. It is therefore preferable to use the Matlab-Compiler to compile your scripts beforehand. We explain more about the Matlab-Compiler as follows.
Matlab-Compiler
There are several options to compile your Matlab code to stand-alone executables/libraries. Being independent of licenses is one of the major advantages here, of course. But when running compiled code with the Matlab Runtime Envirenment (MRE) on MOGON you have to consider the threading of your code just as well as when you run Matlab itself.
mcc Command Arguments
Option | Description | Comment |
---|---|---|
-? | Display help message | Cannot be used in a deploytool app. |
-m | Generate a standalone application. | Equivalent to -W main -T link:exe . Cannot be used in a deploytool app. |
-n | Automatically treat numeric inputs as MATLAB doubles. | Cannot be used in a deploytool app. |
-o outputfile | Specify name of final output file. | Adds appropriate extension. Cannot be used in a deploytool app. |
-R option | Specify run-time options for MATLAB Runtime. | Valid only for standalone applications using MATLAB Compiler.option = -nojvm , -nodisplay , -logfile filename , -startmsg , and -completemsg filename |
-v | Verbose; display compilation steps. |
Single-Threaded
If your code doesn’t need the full multithreading capability, which often is the case, you should compile your code with:
mcc -m my_mfile.m
This causes the -singleCompThread
option to be applied automatically, which limits MATLAB to a single computational thread. This is enabled by default on MOGON.
Using -singleCompThread
also ensures that your standalone code will run on a single computational thread, which not only doesn’t frustrate the core scheduler and the other users less, but also improves the performance of your code by spending less time scheduling all the threads on a core.
Hello MOGON Example
function hellomogon
fprintf('\n======================\n')
fprintf('===[ Hello MOGON! ]===\n')
fprintf('======================\n\n')
N = maxNumCompThreads;
fprintf('\nNumber of Computational Threads: %g\n\n', N)
end
module load math/MATLAB
mcc -m -R -nodisplay mcc_example.m -o hello_mogon_single
./hello_mogon_single
======================
####[ Hello MOGON! ]
======================
Number of Computational Threads: 1
Multi-Threaded
Generally, Matlab detects the number of physical cores and opens the same amount of threads to make full use of the multithreading implemented in the built-in functions. By calling
mcc -m -R -multiCompThread my_mfile.m
you obtain multithread code. Often this might be wanted, but you have to make sure that you select the appropriate resources for this then - namely, the appropriate CPUs. Since Matlab wants to use everything on a host you’ll have to set#SBATCH <nowiki>--</nowiki>cpus-per-task
and an appropriate memory reservation.
Hello MOGON Example
function hellomogon
fprintf('\n======================\n')
fprintf('===[ Hello MOGON! ]===\n')
fprintf('======================\n\n')
N = maxNumCompThreads;
fprintf('\nNumber of Computational Threads: %g\n\n', N)
end
module load math/MATLAB
mcc -m -R -multiCompThread -nodisplay mcc_example.m -o hello_mogon_multi
./hello_mogon_multi
======================
===[ Hello MOGON! ]===
======================
Number of Computational Threads: 20
Submitting a Serial Matlab Job
We would like to explain with a typical Hello MOGON!
example how you can achieve this.
The trivial MATLAB script has only one line:
fprintf('Hello MOGON!<br/>n')
Notice: Save your file with the .m
extension, but call it without the .m
extension.
A serial Job requires only one core, the Slurm job script serial_matlab_job.slurm
therefore would read as follows:
#!/bin/bash
#SBATCH --partition=smp
#SBATCH --account=<YourHPC-Account>
#SBATCH --time=0-00:02:00
#SBATCH --mem-per-cpu=512 #0.5GB
#SBATCH --ntasks=1
#SBATCH --job-name=serial_matlab
#SBATCH --output=%x_%j.out
#SBATCH --error=%x_%j.err
module purge
module load math/MATLAB
matlab -singleCompThread -nodisplay -nosplash -batch hello_mogon
The start-up options (-singleCompThread
, -nodisplay
, -nosplash
) used are explaind above. To run the script, you can now simply submit it to the batch system with the following command:
sbatch serial_matlab_job.slurm
After the job completes, you can view the output with:
cat matlab-example.out
which should give you
[ ... ]
Hello MOGON!
as Matlab will not be able to fully utilize the node, but considers every FPU equal to a CPU.
Submitting a Multi-threaded Matlab Job
The following example is a job that uses Matlab’s Parallel Computing Toolbox. You can benefit from the built-in multithreading provided by Matlab’s BLAS implementation, if your code makes use of this Toolbox or if you have intense computations. The following is an example of MathWorks and can be found here.
slurm_cpus = str2double(getenv('SLURM_CPUS_PER_TASK'));
fprintf('Number of available Slurm CPUs is: %g\n', slurm_cpus);
myCluster = parpool('local',slurm_cpus);
fprintf('The Number of MATLAB Workers is: %g\n', myCluster.NumWorkers);
tic
n = 200;
A = 500;
a = zeros(n);
parfor i = 1:n
a(i) = max(abs(eig(rand(A))));
end
fin = toc;
fprintf('With %g CPUs per Task the calculation took %g seconds.\n', slurm_cpus, fin);
The Slurm job script is kept simple, with one process that has four cores for multithreading:
#!/bin/bash
#SBATCH --account=<YourHPC-Account>
#SBATCH --job-name=parallel_matlab_job
#SBATCH --output=%x_%j.out
#SBATCH --error=%x_%j.err
#SBATCH --partition=smp
#SBATCH --time=0-00:05:00
#SBATCH --mem-per-cpu=4096 #4GB
#SBATCH --ntasks=1
#SBATCH --cpus-per-task=4
module purge
module load math/MATLAB/2018b
matlab -nodisplay -nosplash -batch parfor_matrix
To run the script, simply submit it to the scheduler with the following command:
sbatch parallel_matlab_job.slurm
After the job has finished, view the output with the following command:
cat parallel_matlab*.out
which should give you the following output:
[ ... ]
With 4 CPUs per Task the calculation took 17.0605 seconds.
For optimal performance, the value of --cpus-per-task
must be adjusted. Use the smallest value that gives you a significant performance gain.
The matrix multiplication example from above has the following runtimes on MOGON II with different --cpus-per-task
:
CPUs per Task | Runtime (s) |
---|---|
2 | 29.22 |
4 | 17.06 |
6 | 11.43 |
8 | 8.40 |
JVM
The Parallel Computing Toolbox requires JVM, please do not specify -nojvm
if you want to use it!
Submitting a Matlab Job on GPUs
Testing computationally intensive operationsFor operations where the number of floating-point computations performed per element read from or written to memory is high, the memory speed is much less important. In this case the number and speed of the floating-point units is the limiting factor. These operations are said to have high “computational density”.
A good test of computational performance is a matrix-matrix multiply. For multiplying two $N \times N$ matrices, the total number of floating-point calculations is $$ FLOPS(N) = 2N^3 - N^2 $$
Two input matrices are read and one resulting matrix is written, for a total of $3N^2$ elements read or written. This gives a computational density of $(2N - 1)/3$ FLOP/element. Contrast this with
plus
as used above, which has a computational density of $1/2$ FLOP/element.MATLAB Help Center, Measuring GPU Performance
gpu = gpuDevice();
fprintf('Using an %s GPU\n', gpu.Name)
sizeOfDouble = 8;
sizes = power(2, 12:2:26);
N = sqrt(sizes);
mmTimesHost = inf(size(sizes));
mmTimesGPU = inf(size(sizes));
for ii=1:numel(sizes)
% First do it on the host
A = rand( N(ii), N(ii) );
B = rand( N(ii), N(ii) );
mmTimesHost(ii) = timeit(@() A*B);
% Now on the GPU
A = gpuArray(A);
B = gpuArray(B);
mmTimesGPU(ii) = gputimeit(@() A*B);
end
#!/bin/bash
#SBATCH --account=<YourHPC-Account>
#SBATCH --job-name=matlab_gpu_job
#SBATCH --output=%x_%j.out
#SBATCH --error=%x_%j.err
#SBATCH --partition=m2_gpu
#SBATCH --gres=gpu:1
#SBATCH --time=0-00:35:00
#SBATCH --mem-per-cpu=4096 #4GB
#SBATCH --ntasks=1
#SBATCH --cpus-per-task=4
module purge
module load math/MATLAB/2018b
matlab -nodisplay -nosplash -batch gpu_perf
cat gpu_job_*.out
[ ... ]
Achieved peak calculation rates of 72.9 GFLOPS on Host and 390.7 GFLOPS on GPU
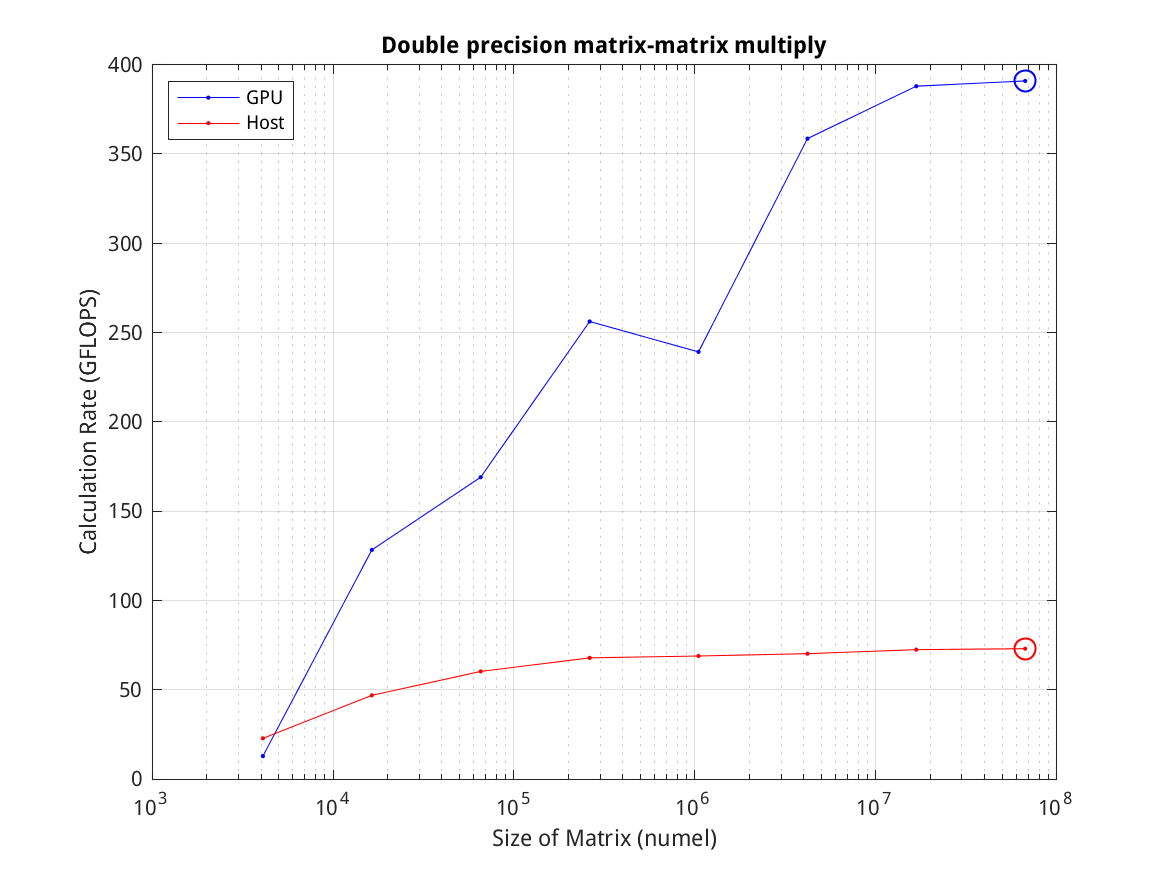
How-To: Compiling an m-file via Job
In the introduction on compiling m-Files in this article, you have probably observed that even the simple Hello MOGON!
-Funcion took its moment to compile into a stand-alone C application. With larger scripts, of course, compile time will not improve, which is why you should always compile m-File in a job on the localscratch directory.
The following is a short, simple example demonstrating how to create such a Job that compiles m-files on the localsratch directory.
Steps
Start by creating a new folder and change to it, e.g.:
mkdir matlabEx && cd matlabEx
You can simple delete the entire folder after working through this How-To.
We will re-use the familiar Hello MOGON!
-script from the previous examples:
function hellomogon
fprintf('\n======================\n')
fprintf('===[ Hello MOGON! ]===\n')
fprintf('======================\n\n')
end
The job script is a little bit more complex. In the first lines, the usual and necessary job options are given. With the line #SBATCH --signal=B:SIGUSR2@60
we send the signal SIGUSR2
to the application one minute before the job reaches its walltime. We then define variables for the current working directory and an ouput folder where we want to save our resulting files. After loading the proper MATLAB module, we define the cleanup function, which simply copies all files from the localscratch directory to the output directory, either after the job completed successfully or one minute before the job reaches its time limit. The subsequent lines only create folders or changes into them. The actual compilation takes place in the line mcc -m ...
already on the localscratch directory of the compute node. Of course, you can provide the compiler with all the options that are given above or the ones you can find in the MATLAB documentation.
#!/bin/bash
#SBATCH --account=<YourHPC-Account>
#SBATCH --partition=smp
#SBATCH --constraint=skylake
#SBATCH --ntasks=1
#SBATCH --cpus-per-task=6
#SBATCH --time=0-00:05:00
#SBATCH --mem=2048
#SBATCH --signal=B:SIGUSR2@60 # e.g. signal 1min before job will end - time is in seconds
# Store working directory to be safe
# Define Input- and Outputfile
SAVEDPWD=$(pwd)
FILENAME=$1
OUTNAME="hello_mogon_compJob"
module load math/MATLAB
# We define a bash function to do the cleaning when the signal is caught
cleanup(){
# Note: The following only works on single with output on the node,
# where the jobscript is running.
# For multinode output, you can use the 'sgather' command or
# get in touch with us, if the case is more complex.
cp -R /localscratch/${SLURM_JOB_ID}/* ${SAVEDPWD}/output &
wait
exit 0
}
# Register the cleanup function when SIGUSR2 is sent,
# one minute before the job gets killed
trap 'cleanup' SIGUSR2
# Create the output directory
mkdir ${SAVEDPWD}/output
# Copy input file
cp ${SAVEDPWD}/$FILENAME /localscratch/${SLURM_JOB_ID}
# Go to jobdir and start the program
cd /localscratch/${SLURM_JOB_ID}
mcc -m -R -multiCompThread -nodisplay $FILENAME -o $OUTNAME
# Call the cleanup function when everything went fine
cleanup
Submit the job as usual with the following command:
sbatch compileFile.sh hello_mogon.m
Our cleanup
function copied all results to the output directory, after the stand-alone application has been created, run it as follows:
./output/hello_mogon_compJob
The output on the command line should look similar like this:
======================
===[ Hello MOGON! ]===
======================